variables(Understanding Variables in Programming)
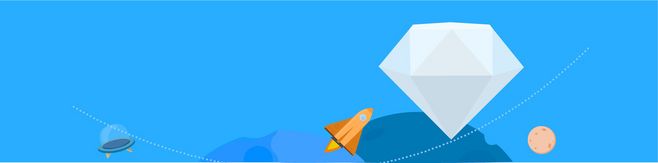
Understanding Variables in Programming
The Basics of Variables
In programming, variables are an important concept that allow developers to store and manipulate data. A variable is like a container or a named storage location that holds a value, which can be changed throughout the program. They play a crucial role in the execution of code, as they enable the processing and modification of data based on predefined rules and algorithms.
Variables can hold different types of data, such as numbers, text, or even complex structures like arrays or objects. Each variable has a unique name and a specific data type, which determines the range of values it can store and the operations that can be performed on it.
Declaring and Assigning Variables
In order to use a variable in a program, it needs to be declared and assigned a value. Declaring a variable involves specifying its name and data type. This tells the computer's memory to allocate space for the variable so that it can be used later in the program. For example, in JavaScript, you can declare a variable using the \"var\", \"let\", or \"const\" keywords:
var age;
This line of code declares a variable named \"age\". However, since no initial value is assigned, the variable's value is considered to be \"undefined\". To assign a value to a variable, you can use the assignment operator \"=\", along with the desired value. For example:
age = 25;
This assigns the value 25 to the variable \"age\". From this point on, the variable \"age\" holds the value 25 until it is changed or reassigned.
Using Variables in Programming
Once a variable is declared and assigned a value, it can be used throughout the program. Variables can be used in various ways, such as performing calculations, storing user input, or controlling program flow. They provide flexibility and allow for dynamic behavior in software applications.
For example, let's consider a simple program that calculates the area of a rectangle based on user input:
<html> <body> <script> var length = parseFloat(prompt(\"Enter the length:\")); var width = parseFloat(prompt(\"Enter the width:\")); var area = length * width; document.write(\"The area of the rectangle is: \" + area); </script> </body></html>
In this program, the variables \"length\" and \"width\" are used to store the user's input, while the variable \"area\" holds the calculated value. The result is then displayed using the \"document.write\" method.
Variables can also be modified or updated as the program executes. For example, you can increment a numeric variable by a certain value, concatenate strings, or modify elements within an array. This flexibility allows for dynamic data manipulation and is essential in creating advanced and interactive applications.
Scoping of Variables
Variables in programming languages have different scopes, which define their accessibility within the program. Scope determines where a variable can be accessed and manipulated. There are generally two types of scope: global scope and local scope.
A variable with global scope can be accessed from anywhere within the program. It is declared outside of any functions or code blocks, making it accessible throughout the entire program. This can be useful for storing data that needs to be shared and accessed across different parts of the program.
On the other hand, variables with local scope are declared within a specific function or code block. They are only accessible within that particular function or block and cannot be accessed from outside. This helps to prevent naming conflicts and allows for encapsulation of data within different parts of the program.
Understanding variable scoping is important in order to avoid potential issues, such as accidentally modifying a variable in one part of the program that affects its value in another part.
Conclusion
Variables are a fundamental concept in programming that enable the storage, manipulation, and retrieval of data. They allow for dynamic behavior and flexibility in software applications, making them an essential component of any programming language. By understanding how to declare, assign, and use variables effectively, developers can create robust and efficient programs that can handle complex data operations.
So, whether you are a beginner learning the basics of programming or an experienced developer looking to enhance your skills, understanding variables is critical to writing clean, efficient, and functional code.