priority_queue(Exploring the Functionality of a Priority Queue in C++)
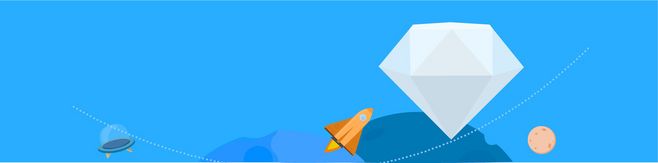
Exploring the Functionality of a Priority Queue in C++
A priority queue is a useful data structure in computer science that allows elements to be stored and retrieved based on their priority. Similar to a regular queue, a priority queue follows the First-In, First-Out (FIFO) principle. However, unlike a regular queue, a priority queue assigns a certain priority value to each element, and the elements with higher priority are dequeued first. In this article, we will explore the functionality of a priority queue in C++ and understand its implementation and applications.
Introduction to Priority Queue
Definition: A priority queue is an abstract data type that is similar to a queue, but instead of operating on a FIFO basis, elements are dequeued based on their priority.
A priority queue stores a collection of elements, each associated with a priority value. The elements in the queue are dynamically sorted according to their priorities. An element with a higher priority value is considered to have a higher priority and is positioned towards the front of the queue.
Operations on a Priority Queue
A priority queue supports the following basic operations:
- Enqueue: This operation inserts an element into the priority queue while preserving the order of priorities.
- Dequeue: This operation removes and returns the element with the highest priority from the priority queue.
- Peek: This operation returns the element with the highest priority without removing it from the priority queue.
Implementing a Priority Queue in C++
In C++, the Standard Template Library (STL) provides a container class called priority_queue
to implement a priority queue. This class is defined in the <queue>
header file and is a part of the collection of container classes.
To use the priority_queue
class, we need to include the <queue>
header and declare a priority queue object. By default, the elements of the priority queue are stored in decreasing order of priority.
Here's an example of how to use the priority_queue
class to implement a priority queue of integers in C++:
#include <queue>#include <iostream>int main() { std::priority_queue<int> pq; pq.push(10); pq.push(30); pq.push(20); while (!pq.empty()) { std::cout << pq.top() << \" \"; pq.pop(); } return 0;}
The above code creates a priority queue pq
using the priority_queue
class. Three integers (10, 30, and 20) are pushed into the queue using the push()
function. The top()
function is used to access the element with the highest priority (in this case, 30), and the pop()
function is used to remove that element from the queue.
Applications of a Priority Queue
Priority queues can be useful in many applications, including but not limited to:
- Task Scheduling: Priority queues can be used in task scheduling algorithms, where tasks with higher priority need to be executed first.
- Graph Algorithms: Priority queues are commonly used in graph algorithms like Dijkstra's algorithm and Prim's algorithm.
- Event-Driven Simulations: Priority queues are helpful in event-driven simulations to ensure that events are processed in the order of their occurrence time.
These are just a few examples of how priority queues can be applied in various fields of computer science and software engineering. The flexibility and efficiency of priority queues make them a valuable tool for solving complex problems that involve prioritization.
In conclusion, a priority queue is a powerful data structure that allows elements to be stored and retrieved based on their priority. In C++, the priority_queue
class of the Standard Template Library provides a convenient way to implement and utilize a priority queue. By understanding the functionality and uses of priority queues, developers can leverage this data structure to enhance the efficiency of their programs and algorithms.