prototypes(Understanding Prototypes in JavaScript)
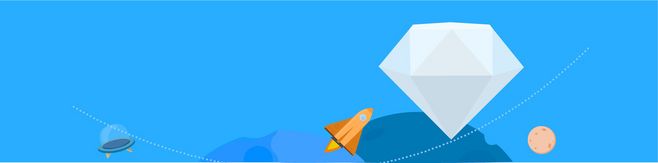
Understanding Prototypes in JavaScript
Introduction to Prototypes
Prototypes play a crucial role in the JavaScript language. They are fundamental to how objects work and are key to understanding inheritance and object-oriented programming in JavaScript. In this article, we will explore prototypes and gain a deeper understanding of how they function.
What are Prototypes?
A prototype in JavaScript is an object from which other objects inherit properties and methods. Every object in JavaScript has a prototype, except for the base object, which is created when you define an object using literal notation or the Object()
constructor. Prototypes are conceptually similar to classes in other programming languages, but in JavaScript, they are more flexible and dynamic.
Using Prototypes for Inheritance
One of the primary uses of prototypes in JavaScript is for inheritance. Through the prototype chain, objects can inherit properties and methods from their prototypes. When you access a property or method on an object, JavaScript first looks for it on the object itself. If it doesn't find it, it continues the search on the object's prototype, and so on, until it reaches the base object.
Creating Prototypes
To create a prototype, you can use the Object.create()
method or by assigning an object literal to the prototype
property of a constructor function. Let's take a look at an example:
In the example above, we define a constructor function Person
with two properties name
and age
. We then add a greet
method to the Person.prototype
object. When we create a new object using the Person
constructor, it inherits the greet
method from the prototype, allowing us to call it on the john
object.
Modifying Prototypes
Prototypes are dynamic, which means you can modify them even after creating objects. If you modify a prototype, all objects created from that prototype will inherit the changes. This flexibility is one of JavaScript's powerful features.
Prototypal Inheritance Patterns
JavaScript supports multiple inheritance patterns using prototypes. You can create a prototype chain by linking objects together, allowing for complex inheritance relationships. Common patterns include the constructor pattern, the prototype pattern, and the combination pattern.
Common Pitfalls and Considerations
While prototypes offer many benefits, there are also some considerations to keep in mind. One common pitfall is accidentally modifying the prototype of a built-in object, such as Array.prototype
or Object.prototype
. This can lead to unexpected behavior and is generally considered bad practice.
Conclusion
Prototypes are a fundamental concept in JavaScript that allows for object inheritance and dynamic modification of objects. Understanding prototypes is crucial for mastering JavaScript and for building efficient and scalable applications. By leveraging the power of prototypes, you can create reusable and extensible code. So, go ahead and harness the power of prototypes in your JavaScript projects!