collections(Introduction to Collections in Python)
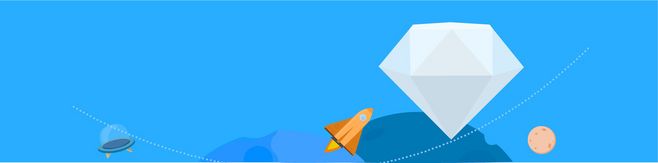
Introduction to Collections in Python
Collections in Python are an essential part of the programming language, providing a way to store and manage groups of related data. They offer a wide range of data structures that are built-in and highly efficient, allowing developers to handle different types of data with ease. In this article, we will explore the various collection modules available in Python and understand their usage and benefits.
The Collections Module
The Python collections module is a built-in module that provides specialized high-performance data structures. It includes alternatives to the built-in data types, such as lists, tuples, and dictionaries, and offers additional data structures like named tuples, deque, defaultdict, and Counter.
Named Tuples: Named tuples are a subclass of tuples and are used to create tuple-like objects that have named fields accessible by attribute lookup. They can be used as lightweight alternatives to define class structures without the need to create a full class.
Deque: A deque, short for \"double-ended queue,\" is an optimized list that allows fast operations from both ends. It offers append and pop operations on either side, making it an efficient choice for implementing queues and stacks.
Defaultdict: A defaultdict is a subclass of the built-in dictionary. It overrides one method and adds another compared to a regular dictionary. The overridden method, __missing__, provides a default value when a non-existent key is accessed. This eliminates the need to check for key existence before accessing or modifying its value.
Counter: A Counter is a dictionary subclass that is used to count hashable objects. It provides a convenient way to keep track of counts for different items, such as occurrences of words in a text or frequencies of elements in a dataset.
Working with Collections
To start using the collections module, you need to import it into your Python script or interactive session:
import collections
Once imported, you can access the different data structures provided by the module. Let's explore their usage with some examples.
Example 1: Named Tuples
Named tuples are commonly used to represent a specific data structure. Let's say we want to store information about a person, such as their name, age, and occupation. We can define a named tuple called \"Person\" with fields corresponding to each attribute:
Person = collections.namedtuple('Person', ['name', 'age', 'occupation'])
We can now create instances of this named tuple and access their values using dot notation:
person1 = Person('John', 25, 'Engineer')
print(person1.name) # Output: John
print(person1.age) # Output: 25
print(person1.occupation) # Output: Engineer
Example 2: Deque
Deque is an excellent choice for implementing a queue or stack data structure. Let's see how we can use a deque as a queue:
queue = collections.deque()
queue.append('item1')
queue.append('item2')
queue.append('item3')
print(queue.popleft()) # Output: item1
print(queue.popleft()) # Output: item2
print(queue.popleft()) # Output: item3
In the above example, we create a deque object called \"queue\" and append items to it using the append() method. To retrieve items from the queue, we use the popleft() method, which removes and returns the leftmost item.
Example 3: Defaultdict
A defaultdict is useful when we want to assign a default value to a nonexistent key. Let's say we want to count the frequency of characters in a string:
string = 'hello'
char_count = collections.defaultdict(int)
for char in string:
char_count[char] += 1
print(char_count) # Output: defaultdict(, {'h': 1, 'e': 1, 'l': 2, 'o': 1})
The defaultdict automatically assigns a default value of 0 (in this case, int) when a new key is encountered. This allows us to increment the count for each character without explicitly checking for key existence.
Example 4: Counter
Counters are extremely useful when we need to count the occurrences of elements in a collection. Let's find the most common words in a text:
text = \"Lorem ipsum dolor sit amet, consectetur adipiscing elit. Sed luctus diam vitae mass.\"
word_count = collections.Counter(text.split())
print(word_count.most_common(3)) # Output: [('Lorem', 1), ('ipsum', 1), ('dolor', 1)]
In the above example, we create a Counter object called \"word_count\" by splitting the given text into words. The most_common() method returns the most common n elements, sorted by their counts in descending order.
Conclusion
The collections module in Python provides powerful data structures that are essential for various programming tasks. Named tuples allow us to create lightweight data structures, while deques offer efficient operations for implementing queues and stacks. Defaultdict eliminates the need for checking key existence, and Counters provide a convenient way to count occurrences. These data structures, along with others available in the collections module, make Python a versatile language for handling and managing collections of data.