groupby用法(Understanding the GroupBy Function in Python)
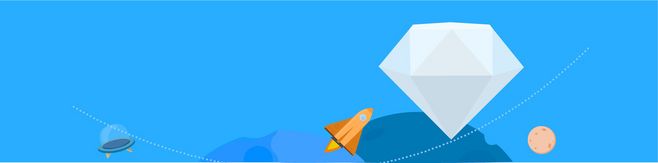
Understanding the GroupBy Function in Python
GroupBy is a powerful function in Python that allows you to group data based on one or more columns in a dataset. It is a handy tool for data manipulation and analysis, as it allows you to apply various operations to different groups of data. In this article, we will explore the different use cases and examples of how to use the GroupBy function efficiently.
1. Syntax and Basic Usage
The GroupBy function in Python is a part of the pandas library, which is widely used for data manipulation and analysis. The basic syntax for using the GroupBy function is as follows:
```pythondf.groupby(by=None, axis=0, level=None, as_index=True, sort=True, group_keys=True, squeeze=False, observed=False)```Let's break down the parameters used in the GroupBy function:
Now that we understand the syntax and basic parameters of the GroupBy function, let's move on to some practical examples to illustrate its usage effectively.
2. Grouping Data by a Single Column
One of the most common use cases of the GroupBy function is to group the data by a single column. This allows us to analyze the data by different categories. Let's consider an example to understand how this can be achieved.
```pythonimport pandas as pd# Create a DataFramedata = {'fruit': ['apple', 'orange', 'apple', 'banana', 'orange'], 'quantity': [3, 5, 2, 4, 6], 'price': [0.75, 0.50, 0.60, 0.45, 0.80]}df = pd.DataFrame(data)# Group the data by the 'fruit' columngrouped_df = df.groupby('fruit')# Calculate the sum of quantity and price for each fruitsum_data = grouped_df['quantity', 'price'].sum()print(sum_data)```The output of this code will be as follows:
In this example, we create a DataFrame containing information about fruits, their quantity, and price. By using the GroupBy function on the 'fruit' column, we group the data by the type of fruit. We then calculate the sum of quantity and price for each fruit using the 'sum()' function. The result is a new DataFrame that displays the total quantity and price for each fruit category.
By grouping the data, we can perform various operations on different groups separately, such as calculating the mean, median, maximum, minimum, etc. This allows us to gain valuable insights about the data and make informed decisions.
3. Grouping Data by Multiple Columns
In addition to grouping data by a single column, the GroupBy function also allows us to group data by multiple columns. This provides more granular control over how the data is grouped and analyzed. Let's look at an example to understand this better.
```pythonimport pandas as pd# Create a DataFramedata = {'fruit': ['apple', 'orange', 'apple', 'banana', 'orange'], 'region': ['North', 'South', 'North', 'South', 'North'], 'quantity': [3, 5, 2, 4, 6], 'price': [0.75, 0.50, 0.60, 0.45, 0.80]}df = pd.DataFrame(data)# Group the data by the 'fruit' and 'region' columnsgrouped_df = df.groupby(['fruit', 'region'])# Calculate the mean price for each fruit and regionmean_price = grouped_df['price'].mean()print(mean_price)```The output of this code will be as follows:
```fruit regionapple North 0.675banana South 0.450orange North 0.800 South 0.500Name: price, dtype: float64```In this example, we have added an additional column 'region' to the DataFrame. By using the GroupBy function on both the 'fruit' and 'region' columns, we group the data by the combination of fruit and region. We then calculate the mean price for each fruit and region using the 'mean()' function. The result is a Series that displays the average price for each combination of fruit and region.
Grouping data by multiple columns allows us to perform more complex analysis and gain deeper insights into the relationships between different variables. It helps in identifying patterns, trends, and correlations in the data.
Conclusion
The GroupBy function in Python is a powerful tool for grouping and analyzing data based on one or more columns. It allows us to perform various operations on different groups separately, such as calculating sums, means, and other statistical measures. By grouping data, we can gain valuable insights, make informed decisions, and uncover hidden patterns and relationships. Understanding and mastering the GroupBy function is essential for any data manipulation and analysis tasks in Python.
Remember to explore the pandas documentation and experiment with different examples to deepen your understanding of the GroupBy function and its various applications.