parentelement(Understanding the parentElement property in JavaScript)
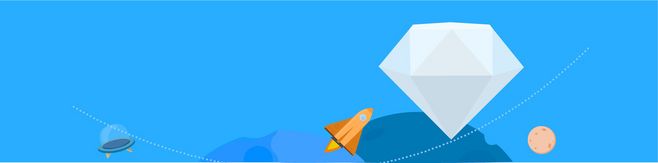
Understanding the parentElement property in JavaScript
Introduction
In JavaScript, the parentElement property is used to access the parent element of a specific HTML element. It allows developers to navigate the HTML document tree and perform various operations on parent elements. This article will explore the parentElement property in depth, explaining its usage and providing examples to illustrate its functionality.
Usage of parentElement
The parentElement property is primarily used to access the parent element of an HTML element. It returns the parent element as an object, allowing developers to manipulate or retrieve information from it. To use the parentElement property, you need to have a reference to the specific HTML element you want to access the parent of.
Example:
Consider the following HTML structure:
<div id=\"parent\"> <p id=\"child\">This is a child element</p></div>
To access the parent element of the `
` element with the id \"child\", you can use the parentElement property as follows:
const childElement = document.getElementById(\"child\");const parentElement = childElement.parentElement;
Here, `childElement` represents the `
` element with the id \"child\". By calling the `parentElement` property on `childElement`, we get the parent element, which is the `
Manipulating Parent Elements
The parentElement property can be used to manipulate parent elements in various ways. For example, you can change the style or modify the content of the parent element.
Example: Changing the style of the parent element>
const parentElement = document.getElementById(\"parent\");parentElement.style.backgroundColor = \"blue\";
In this example, we access the parent element with the id \"parent\" and change its background color to blue.
Example: Modifying the content of the parent element>
const parentElement = document.getElementById(\"parent\");parentElement.innerHTML = \"Modified parent element\";
In this example, we change the content of the parent element by modifying its innerHTML property. The text \"Modified parent element\" will be displayed instead of the previous content.
Traversing the HTML Document Tree
The parentElement property is particularly useful when traversing the HTML document tree. It allows you to navigate from a child element to its parent element, or even higher levels in the tree.
Example:
<!DOCTYPE html><html><head><title>Example</title></head><body><div id=\"grandparent\"> <div id=\"parent\"> <p id=\"child\">This is a child element</p> </div></div></body></html>
In this example, we have a hierarchy of elements: 'grandparent' is the outermost `
const childElement = document.getElementById(\"child\");const parentElement = childElement.parentElement; // 'parent' elementconst grandparentElement = parentElement.parentElement; // 'grandparent' element
By calling the parentElement property multiple times, we can navigate up the hierarchy of elements and access the desired parent elements.
Conclusion
The parentElement property is a powerful tool that allows developers to access and manipulate parent elements in the HTML document tree. It simplifies the process of navigating through the document structure and enables various operations on parent elements. Understanding and utilizing the parentElement property can greatly enhance the functionality and flexibility of JavaScript code when working with HTML elements.
By leveraging the parentElement property, developers can create dynamic and interactive web applications that seamlessly interact with the HTML document structure.