dictionaries(Understanding Dictionaries in Python)
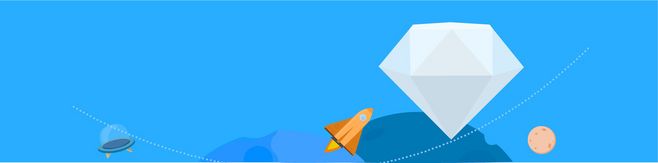
Understanding Dictionaries in Python
Introduction to Dictionaries:
Dictionaries are a fundamental data structure in Python that allow you to store and retrieve data using key-value pairs. While lists and tuples store data in a linear and ordered manner, dictionaries provide a more flexible way of organizing and accessing information. In this article, we will explore the concept of dictionaries, how to create and manipulate them, and why they are widely used in Python programming.
Creating and Accessing Dictionary Elements:
One of the key features of dictionaries is their ability to store and retrieve data using unique keys. Each key in a dictionary is associated with a value, and you can access the value by referencing its key. To create a dictionary, you enclose a comma-separated list of key-value pairs within curly braces, with a colon separating each key and its respective value. Let's look at an example:
```pythonmy_dict = { \"name\": \"John\", \"age\": 25, \"city\": \"New York\"}```You can access the values in a dictionary using the corresponding keys. For example, to access the value associated with the key \"name\" in the above dictionary, you can use the following code:
Modifying and Adding Dictionary Elements:
Dictionaries are mutable, which means you can modify and add elements to them. To modify the value of an existing key, you simply assign a new value to it. For example, let's say we want to change the age of the person in the dictionary we created earlier:
If the key does not exist and you want to add it to the dictionary, you can assign a value to it using the assignment operator. Let's add a new key-value pair for the person's occupation:
```pythonmy_dict[\"occupation\"] = \"Engineer\"```Dictionary Methods and Operations:
Python provides a variety of methods and operations to work with dictionaries efficiently. Some of the commonly used methods include:
keys()
: This method returns a list of all the keys in the dictionary.values()
: This method returns a list of all the values in the dictionary.items()
: This method returns a list of tuples, where each tuple represents a key-value pair in the dictionary.get(key)
: This method returns the value associated with the specified key. If the key does not exist, it returns a default value (which isNone
by default).pop(key)
: This method removes and returns the value associated with the specified key.
In addition to these methods, you can also perform operations like checking if a key exists in a dictionary using the in
keyword, and finding the length of a dictionary using the len()
function.
Conclusion:
Dictionaries are a versatile and powerful data structure in Python that offer a flexible way of organizing and retrieving data using key-value pairs. Their ability to store and access elements in an unordered and efficient manner makes them a fundamental tool for various programming tasks. By understanding the basics of dictionaries and their associated methods, you can harness their potential and write more clean and efficient Python code.