critical_section(Understanding Critical Section in Programming)
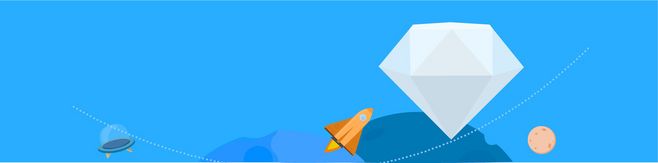
Understanding Critical Section in Programming
The concept of a critical section in programming is essential for ensuring the proper execution of concurrent programs. In this article, we will explore what a critical section is, why it is necessary, and how it can be implemented effectively.
What is a Critical Section?
A critical section refers to a portion of a program where shared resources are accessed or modified by multiple concurrent processes or threads. It is crucial to ensure that only one process or thread can access the shared resource(s) at any given time to prevent data inconsistencies or race conditions.
To understand the need for a critical section, let's consider an example scenario. Suppose we have multiple threads in a program that need to access and update a shared variable simultaneously. In the absence of a critical section, two or more threads might try to modify the value of the shared variable simultaneously, leading to an unpredictable outcome. This can result in incorrect calculations, corrupted data, or program crashes.
Why is a Critical Section Necessary?
A critical section is necessary to maintain data integrity and avoid conflicts when multiple threads or processes access shared resources in a concurrent program. Without proper synchronization mechanisms, such as a critical section, there is no guarantee of the order in which the threads will access or modify shared data.
By defining a critical section, we establish a mutually exclusive region where only one thread can execute at a time. In other words, when a thread enters a critical section, it gains exclusive access to the shared resource(s), preventing other threads from interfering until it completes its execution and leaves the critical section. This ensures that each thread can safely access and modify the shared data without interference from others.
Moreover, a critical section also allows us to impose specific ordering constraints on the execution of threads. For example, we can ensure that a certain thread completes its execution before another thread starts accessing the shared resource(s). This control over the execution order is crucial for achieving the desired behavior and correctness in a concurrent program.
Implementing Critical Section
There are various synchronization mechanisms and techniques that can be used to implement a critical section. One commonly used approach is the use of locks or mutexes (mutual exclusions). A lock can be viewed as a binary semaphore that allows only one thread to acquire it at a time. When a thread enters a critical section, it acquires the lock, and other threads attempting to enter the same critical section are blocked until the lock is released by the thread currently holding it.
Another approach is the use of atomic operations or atomic variables provided by the programming language or library. Atomic operations ensure that specific operations or sequences of instructions are executed atomically, which means there is no possibility of interference or interruption from other threads between these operations.
It is important to note that the implementation of a critical section should be carefully designed and tested to avoid potential issues such as deadlocks or livelocks. Deadlocks occur when threads are waiting indefinitely for resources that will never become available, while livelocks occur when threads are constantly changing their states without making progress.
In addition to locks and atomic operations, there are also more advanced synchronization mechanisms available, such as semaphores, condition variables, and monitors. These mechanisms provide additional flexibility and control over thread synchronization, depending on the requirements of the program.
In conclusion, a critical section plays a vital role in concurrent programming by ensuring that shared resources are accessed and modified in a controlled and synchronized manner. It helps to prevent data inconsistencies, race conditions, and conflicts between multiple threads or processes. By implementing proper synchronization mechanisms, such as locks or atomic operations, we can effectively define and manage critical sections, leading to correct and predictable execution of concurrent programs.