gocheck(Gocheck A Powerful Testing Framework for Go)
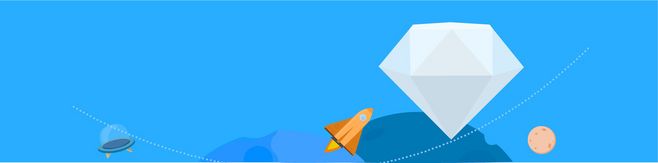
Gocheck: A Powerful Testing Framework for Go
Introduction
Go is a popular programming language known for its simplicity, efficiency, and robustness. It has gained significant traction in the software development community, especially for building system-level software. With the increasing complexity of software systems, automated testing plays a critical role in maintaining code quality. This article introduces Gocheck, a powerful testing framework for Go, and discusses its features and benefits.
Why Gocheck?
Testing is an essential aspect of software development as it ensures that the code behaves as expected and helps catch bugs early in the development cycle. Gocheck provides a comprehensive and intuitive testing framework for Go, making it easier for developers to write effective tests. Some key reasons to use Gocheck are:
- Simplicity: Gocheck follows the principle of simplicity, with a straightforward API that is easy to understand and use. It allows developers to write concise and readable tests, contributing to maintainable code.
- Rich Asserts: Gocheck offers a wide range of assertion functions, making it effortless to write test cases with various conditions. It provides functions to check for equality, inequality, nil values, error handling, and more, enabling thorough testing.
- Parallel Execution: Gocheck supports parallel execution of tests by running them concurrently, utilizing the available system resources efficiently. This feature speeds up the overall testing process, especially when dealing with a large number of test cases.
- Fixture Support: Gocheck allows the setup and tear down of test fixtures using the
SetUpTest
andTearDownTest
methods. This enables the initialization of common test data and cleanup after tests, facilitating test organization and reducing redundant code. - Benchmarking: Gocheck supports benchmark testing, allowing developers to measure the performance of their code and identify potential bottlenecks. This feature is valuable for optimizing critical sections of code to ensure efficient execution.
Getting Started with Gocheck
To start using Gocheck, you need to install it as a dependency in your Go project. You can install it using the Go command:
go get gopkg.in/check.v1
Once installed, you can import the Gocheck package in your test files and start writing tests. Create a new file, e.g., mytest_test.go
, and include the following import statements:
import ( \"testing\" . \"gopkg.in/check.v1\")
Gocheck uses the built-in testing
package from Go and extends it with additional functionality. Tests written with Gocheck need to be defined in test suites that satisfy the Gocheck interface. For example, you can define a test suite for your package as follows:
type MyTestSuite struct{}var _ = Suite(&MyTestSuite{})
Next, you can start writing test functions within the test suite. These functions should begin with the word \"Test\" and accept a single parameter of type *C
, which is the test context provided by Gocheck. For example:
func (s *MyTestSuite) TestAddition(c *C) { result := Add(2, 3) c.Assert(result, Equals, 5)}
In the above example, the c.Assert()
function is used to assert that the result of the addition operation is equal to 5. Gocheck provides various assertion functions like c.Assert()
to assert different conditions in the tests.
After writing the test functions, you can run the tests using the go test
command in your project directory:
go test
Gocheck will discover and execute all the test functions defined in the test files. The test results will be displayed in the console, indicating whether the tests passed or failed.
Conclusion
Gocheck is a powerful testing framework for Go that simplifies and enhances the testing process. With its intuitive API, rich assertion functions, and support for parallel execution and fixtures, Gocheck empowers developers to write effective tests and ensure code quality. By adopting Gocheck as your testing framework, you can establish a robust testing culture and deliver high-quality software with confidence.
Give Gocheck a try in your next Go project and experience the benefits it brings to your testing workflow!