asynctask(Exploring the Power of Asynctask in Android Development)
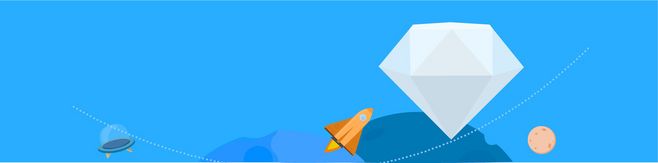
Exploring the Power of Asynctask in Android Development
Android app development requires the handling of many complex tasks that can often impact the user experience. As your application begins to encompass more complex features, it’s vital to ensure it remains responsive and performs efficiently. One solution available to developers that can help improve the responsiveness of their applications is the use of AsyncTask.
What is AsyncTask?
AsyncTask is a class provided by the Android SDK that enables developers to run operations that run independently of the user interface (UI) thread. Specifically, it is designed to handle tasks that involve accessing resources that may take some time to process, such as network or database operations, or simply running tasks that might cause UI blocking. Using AsyncTask enables such heavy tasks to run in the background, freeing the UI thread to remain responsive and handle user interaction without any lag.
AsyncTask is easy to use and can be a powerful tool to elevate your Android app’s user experience. Here’s how to utilize AsyncTask to maximize its benefits:
How to Use AsyncTask in Your Application
The AsyncTask class makes it easy for developers to offload processing tasks into a separate thread that won't block the UI. To use it, you first need to create your internal subclass of the AsyncTask class, which includes an implementation of the doInBackground() callback method that specifies the code to run on a separate thread. Once you specify the appropriate parameters and call the AsyncTask subclass from the UI thread, the execution of the doInBackground() method starts in the background thread.
Here is the skeleton code for creating an AsyncTask:
```java private class MyAsyncTask extends AsyncTaskHere, the `doInBackground` method carries out the work of your task in the background, while the `onPostExecute` method is executed once the background work is complete and the result is passed back to the UI thread.
Best Practices and Considerations
There are some best practices that you should keep in mind when using AsyncTask in your application:
- Only use AsyncTask for short-duration operations.
- Avoid using AsyncTask for long-running tasks such as data syncing or media downloading.
- Use AsyncTask in conjunction with other threading mechanisms, such as ThreadPoolExecutor or Service.
- Be mindful of memory leaks that can occur if your AsyncTask instance is still referenced by the UI thread after configuration changes, such as screen rotation or app backgrounding and reopening.
By following best practices and taking special considerations when working with AsyncTask, you can take full advantage of the power of asynchronous programming to improve your app’s responsiveness and enhance user experience.
Conclusion
In this article, we’ve explored the power of AsyncTask and its ability to help Android developers handle heavy tasks easier and more efficiently. By taking advantage of asynchronous programming in your application, you can improve the user experience of your app dramatically, especially for operations that might require blocking the UI thread. Remember to always follow the best practices and guidelines to avoid potential pitfalls and ensure your app runs smoothly. With a little extra effort, your users will surely appreciate the results!