dialogresult(Understanding and Utilizing the DialogResult in C#)
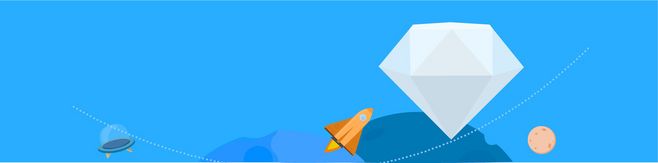
Understanding and Utilizing the DialogResult in C#
The DialogResult is an important concept in C# programming that allows for interaction between different forms or dialog boxes in a Windows Forms application. It is used to determine the outcome of a user's interaction with a dialog box and can be utilized to control the flow of the program based on the user's choices. This article will provide an in-depth understanding of the DialogResult and demonstrate its practical usage in C#.
1. What is DialogResult?
The DialogResult is an enumeration in C# that represents the possible results of a dialog box. It consists of predefined values such as \"OK\", \"Cancel\", \"Yes\", \"No\", and others. When a user interacts with a dialog box, such as clicking a button, a DialogResult value is set based on the user's action.
For example, if a user clicks the \"OK\" button on a dialog box, the DialogResult property of that button is set to \"OK\". Similarly, if the user clicks the \"Cancel\" button, the DialogResult property will be set to \"Cancel\". This value can then be accessed and used to perform specific actions based on the user's choice.
2. Using DialogResult in Windows Forms Applications
In a Windows Forms application, the DialogResult can be used to control the flow of the program based on the user's interaction with different forms or dialog boxes. By setting the DialogResult property of buttons or other controls within a dialog box, we can determine what action should be taken when the user interacts with them.
For example, let's consider a scenario where a user needs to confirm their intention to delete a file. When the user clicks the \"Delete\" button, a confirmation dialog box can be displayed with \"Yes\" and \"No\" buttons. By setting the DialogResult property of the \"Yes\" button to \"Yes\" and the \"No\" button to \"No\", we can access the DialogResult value of the clicked button and perform the appropriate action based on the user's choice.
In code, this can be achieved by handling the button click event and checking the DialogResult value. For example:
```csharpprivate void deleteButton_Click(object sender, EventArgs e){ DialogResult result = MessageBox.Show(\"Are you sure you want to delete this file?\", \"Confirmation\", MessageBoxButtons.YesNo); if(result == DialogResult.Yes) { // Perform delete operation } else { // Cancel delete operation }}```3. Customizing DialogResult in Your Application
While the DialogResult enumeration provides a set of predefined values, it is also possible to customize the DialogResult according to the needs of your application. This can be accomplished by creating your own enumeration and assigning custom values to it.
For example, let's say you have a form that collects user preferences, and you want to have a \"Save and Close\" button. By creating a custom DialogResult enumeration with a value of \"SaveAndClose\", you can set the DialogResult property of the \"Save and Close\" button to this custom value. This allows you to handle the button click event and perform specific actions based on this custom DialogResult value.
Here is an example of how you can create and use a custom DialogResult:
```csharppublic enum CustomDialogResult{ SaveAndClose, Cancel, DiscardChanges}private void saveAndCloseButton_Click(object sender, EventArgs e){ this.DialogResult = (DialogResult)CustomDialogResult.SaveAndClose; this.Close();}private void cancelButton_Click(object sender, EventArgs e){ this.DialogResult = (DialogResult)CustomDialogResult.Cancel; this.Close();}```By using a custom DialogResult, you can add more flexibility and customization to the dialog boxes in your application, allowing for a better user experience.
In conclusion, the DialogResult is a fundamental concept in C# programming that enables interaction between different forms or dialog boxes in a Windows Forms application. It allows you to control the flow of the program based on the user's choices and perform specific actions accordingly. By understanding and utilizing the DialogResult effectively, you can create more intuitive and user-friendly applications.